GraphQL in 5 Mins
Learn about GraphQL & build your app supporting GraphQL in just 5 mins
With the increasing complexity of software solutions being developed, software engineers are developing more and more efficient and clever solutions for communication between these systems. GraphQL is one such solution developed by engineers at Facebook and released to the public in 2015 to remove the various weakness of a traditional Rest architecture.
In this article, we will learn to create a simple GraphQL Application that can help you have a hands-on with GraphQL or if you want to build a new application using graphql this can be a good starting point for your solution.
A brief introduction to GraphQL?
GraphQL stands for Graph Query Language, unlike the traditional Query languages graphic does not directly interact directly with a database. But GraphQL defines the contracts through which client interacts the with the API server. In simple words, GraphQL is a query language for your API and a server-side runtime for executing queries using a type system you define for your data. GraphQL isn’t tied to any specific database or storage engine and is instead backed by your existing code and data.
After a brief introduction to graphql, let us come to the topic of this article building a graphql app. For this app, we will use a Springboot app with Kotlin. There are various great dependencies available for the graphql that we can use. but we will focus on Expedia's graphql library which is built upon graphql-java and in my opinion is one of the best libraries that you can use to create a graphql application. The main advantage of the Expedia library is that it allows you to focus on writing your logic abstracting out all the manual tasks of creating graphql schemas manually.
Before starting with the steps I would recommend you to download JDK 11+ and Apache maven as prerequisites to the app.
Steps for GraphQL App
Step 1:
Download the app using spring initializer. For this app, we will be using the Spring Reactive Web since the graphql-kotlin-spring-server library works on Spring Reactive Web. For the other options that need to be selected, you can refer to the following screenshot. After providing all the options, just click on the generate button to have your own spring boot app ready for further development.
Step 2:
After downloading the app using spring initializer next step would be importing the same in your IDE. On the first import, it might take some time to download all the dependencies for the project. After all the dependencies are downloaded you can try running the app by running the main class, in our case, it would be MyFirstGraphQLAppApplication.kt. The app should be able to run the app without any error. It will start a netty server which is an inbuilt server for Spring Reactive Web.
Step 3:
As the next step is to add graphql-kotlin-spring-server in-app. Add the following dependency to the pom.xml file. After adding the dependency just run mvn install in the terminal which will download the dependency. Here we are using the 6.0.0 version for the dependency but you can use any latest version available at the time of reading this article.
<dependency>
<groupId>com.expediagroup</groupId>
<artifactId>graphql-kotlin-spring-server</artifactId>
<version>6.0.0</version>
</dependency>
Step 4:
Now we are ready for writing the first graphQL query. Before starting with the queries let us create a separate folder and a file for queries. Create a new folder and file queries>SampleQueries.kt. As the next step add the following code to SampleQueries.kt file.
import com.expediagroup.graphql.server.operations.Query
import org.springframework.stereotype.Component
@Component
class SampleQueries: Query { @GraphQLDescription("My first query")
fun myFirstGraphQLQuery():String{
return "This is my first graphql query.";
}
}
The above code contains a simple function that will return a simple string. But if you try running the code as it is you will get an error due to a missing package, to resolve that add the following property to the application.property. This is required by kotlin graphql library.
graphql.packages=com.example.myFirstGraphQLApp.queries
Try running the app again after the above changes. It should run fine without any errors. After your application is running fine you can try the query in a graphql playground what do you have to do for that…? Nothing. The Kotlin graphql library comes with the inbuilt Prisma labs playground that can be accessed here.
{
myFirstGraphQLQuery
}
How does it work? Expedia GraphQL library will scan the packages for the Query files. For a class to be Query we need to implement the Query interface. The library will generate all the schemas and queries based on the function inside this class.
For a more complex example you can use them for the following code:
Data class for Person:
package com.example.myFirstGraphQLApp.schema
data class Person(
val id: Int,
val firstname:String,
val lastname: String,
val age: Int,
val gender: String,
) {
}
Query to get info:
package com.example.myFirstGraphQLApp.queries
import com.example.myFirstGraphQLApp.schema.Person
import com.expediagroup.graphql.generator.annotations.GraphQLDescription
import com.expediagroup.graphql.server.operations.Query
import org.springframework.stereotype.Component
@Component
class SampleQueries: Query {
@GraphQLDescription("Getting Person's Info")
fun getPersonInfo(): Person{
return Person(
id = 1,
firstname = "Jhonny",
lastname = "Parker",
age = 35,
gender = "Male"
)
}
}
application.properties:
graphql.packages=com.example.myFirstGraphQLApp.queries, com.example.myFirstGraphQLApp.schema
Query:
{
getPersonInfo{
id
firstname
lastname
age
gender
}
}
we can see all the generated schemas under the schemas menu in the playground.
What’s next?
Here in this article, we have scratched the surface of what all we can do using the kotlin graphql library. It provides you loads of features that you can easily use to customize your app and were out of the scope of the article. If you are interested leave a comment and create another article with different features and hands-on examples. Also if you have an existing app you can import the same as a dependency to this app and use that to create complex queries.
Summary
In this article, we learned briefly about graphql. We also looked at steps to create your own first, simple graphql app. Hope this will give you a good runway to get started with the graphql and explore more.
If you loved my work please like and share this article( it’s free :)). Also, do follow me for more articles like these.
Also, check out my other articles:

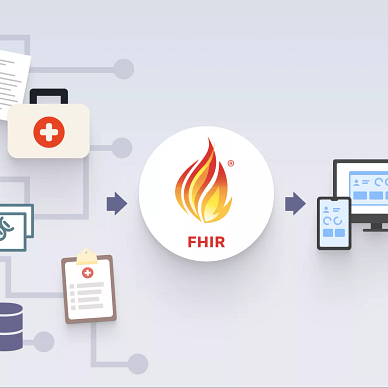
